Futures Exchange Sessions 3.0
This TradingView indicator is the ultimate conclusion to the Futures Exchange Sessions 2.0 indicator. In version 3.0 the user gets full control of the start and end times of three separate dynamic boxes and one horizontal line. If the user wants to visually keep track of killzones, lunches, or any other time span in a trading day, version 3.0 will dynamically expand and keep track of price within the time specified by the user.
Inputs and Style
Everything about the three dynamic boxes and one horizontal line can but independently configured. Color, style, border, width can all be adjusted. In the Settings each box has a text box so the user can give each one a unique name.
Timezone
All of the start and end times are in EST. Additionally, each box and line need a dependent start of each day. This is controlled by a setting where the user can specify a timezone called Start Day Timezone which would be midnight of the respective timezone. In general if a box or line resides within a particular Session pick the corresponding timezone. If the users box/line fits in the Asian Session then choose Asia/Shanghai. If the box/line is within the London Session then choose Europe/London. And the same goes for the New York Session.
Special Notes
- If start time is within one period of the Start Day Timezone in the Settings, then the line/box wonβt display
- Boxes and time lines only display when timeframe is <= 30 minute
- To turn off box text label set opacity to 0%
Screenshots
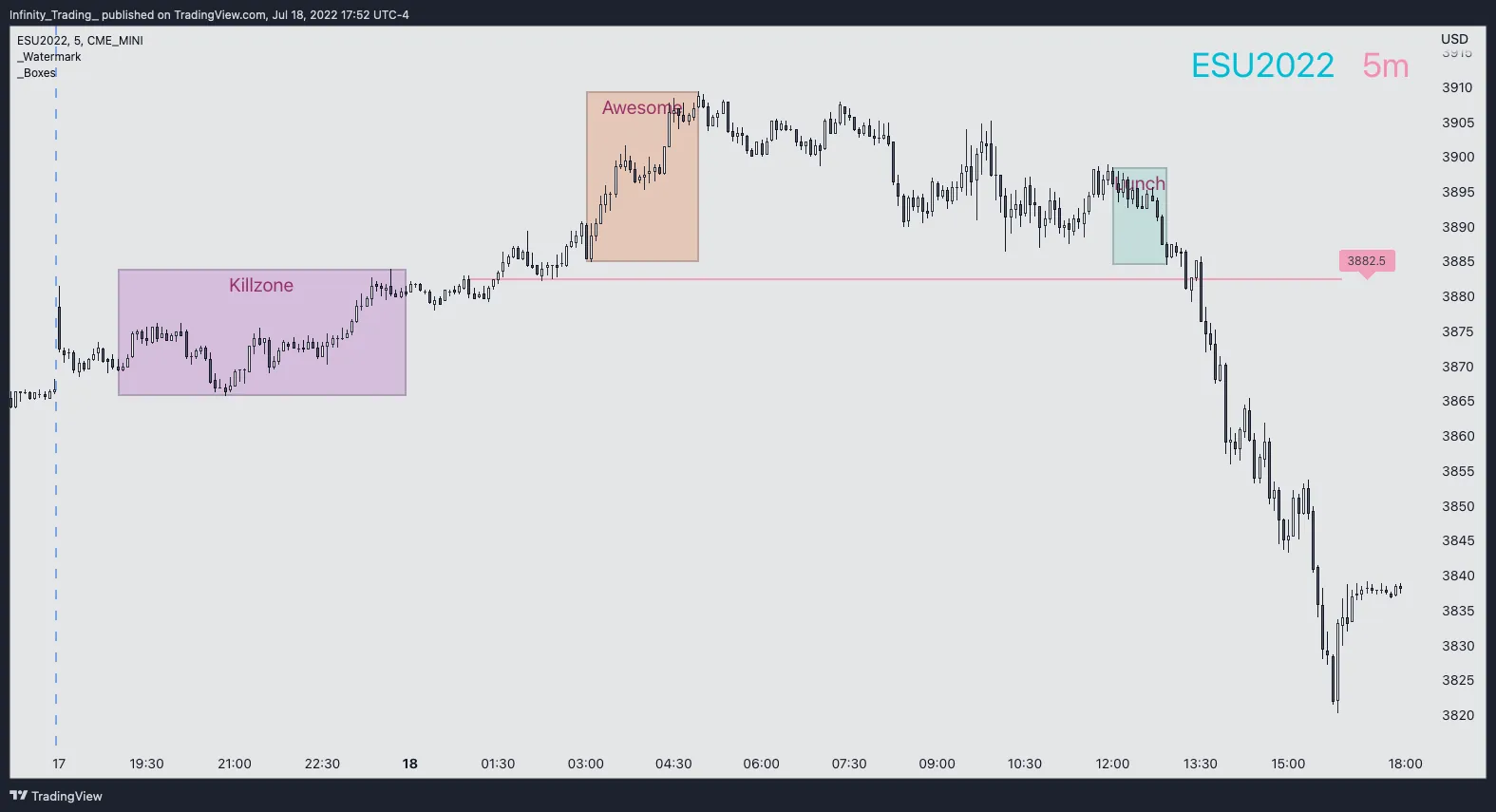
Futures Sessions 3.0 TradingView Indicator
Code
// Β© Infinity_Trading_
// Last Modified: 7/18/2022
//@version=5
indicator(title="Futures Exchange Sessions 3.0", shorttitle="_Boxes", overlay=true, max_boxes_count=200)
// Purpose: Allows the user full control over the start and end times of three separate dynamic time boxes. Also, the user has the ability to set the time
// start and end of a horizontal time line (if the end time is in the future, the line goes to the current bar).
// Usage: Set the start and end times of each of the dynamic boxes. Set the text label of each individual box.
// Choose any time for the horizontal time line.
// The boxes can be customized by fill color, border color, border width, border style, or toggled on/off.
// The box text can be changed by color, size, vertical alignment, or horizontal alignment.
// Notes:
// To turn off box text label set opacity to 0%
// Boxes and time lines only display when timeframe is <= 30 minute
// If start time is within one period of the Start Day Timezone in the Settings, then the line/box won't display
// https://www.tradingview.com/pine-script-reference/
///////////////////////////////////////////////////////////////////////////////// INPUTS
//------------------------------------
// CONTANTS
//------------------------------------
c_milliseconds_in_day = 24 * 60 * 60 * 1000
c_asian_killzone_est = '1900-2359'
c_london_killzone_est = '0300-0459'
c_new_york_killzone_est = '0700-0959'
//------------------------------------
// Boxes
//------------------------------------
c_tooltip_1 = "If the user increments/decrements this integer field and nothing happens, that is because the user is moving throught a weekend or holiday. Please continue incrementing/decrementing and after 2-3 clicks the user will see backgrounds/labels appear or disappear. The algorithm for Days Back is counting every 24-hour period which is why this behavior occurs."
c_tooltip_2 = "To make the boxes/lines start over each day the code must know when to start the day. Since the user is entering times a static start time won't work. Use midnight in the three timezone options as the starting point. If the users box/line fits in the Asian Session then choose Asia/Shanghai. If the box/line is within the London Session then choose Europe/London. And the same goes for the New York Session."
c_tooltip_3 = "Please specify a start & end time within the same 'day' where the 'day' starts at midnight of the timezone selected by the user in the dropdown Start Day Timezone"
var string GDTB = "Dynamic Time Boxes"
i_display_days_back = input.int(defval=15, minval=1, maxval=80, title="Display Only π’ Days Back", group=GDTB, tooltip=c_tooltip_1)
var string G1 = "First Box"
i_1st_box_toggle = input.bool(defval=false, title="First Box On/Offββββ", group=G1, inline="first_toggle")
i_1st_box_bgcolor = input.color(defval=color.new(#e65100, 80), title="Fill Color", group=G1, inline="first_toggle")
i_1st_box_start_day = input.string(defval="Asia/Shanghai", title="Start Day Timezone", options=["America/New_York", "Europe/London", "Asia/Shanghai"], group=G1, tooltip=c_tooltip_2)
i_1st_box_session = input.session(defval=c_asian_killzone_est, title="Box Session Times", group=G1, tooltip=c_tooltip_3)
i_1st_box_text = input.string(defval="1st Box", title="Box Text Label", group=G1)
i_1st_box_border_color = input.color(defval=color.new(#131722, 80), title="Borderβββββββ", group=G1, inline="first_border")
i_1st_box_border_width = input.int(defval=2, title="", minval=1, maxval=20, group=G1, inline="first_border")
i_1st_box_border_style = input.string(defval="solid (β)", title="", options=["solid (β)", "dotted (β)", "dashed (β)"], group=G1, inline="first_border")
var string G2 = "Second Box"
i_2nd_box_toggle = input.bool(defval=false, title="Second Box On/Offβββ", group=G2, inline="second_toggle")
i_2nd_box_bgcolor = input.color(defval=color.new(#f06292, 80), title="Fill Color", group=G2, inline="second_toggle")
i_2nd_box_start_day = input.string(defval="Europe/London", title="Start Day Timezone", options=["America/New_York", "Europe/London", "Asia/Shanghai"], group=G2, tooltip=c_tooltip_2)
i_2nd_box_session = input.session(defval=c_london_killzone_est, title="Box Session Times", group=G2, tooltip=c_tooltip_3)
i_2nd_box_text = input.string(defval="2nd Box", title="Box Text Label", group=G2)
i_2nd_box_border_color = input.color(defval=color.new(#131722, 80), title="Borderβββββββ", group=G2, inline="second_border")
i_2nd_box_border_width = input.int(defval=2, title="", minval=1, maxval=20, group=G2, inline="second_border")
i_2nd_box_border_style = input.string(defval="solid (β)", title="", options=["solid (β)", "dotted (β)", "dashed (β)"], group=G2, inline="second_border")
var string G3 = "Third Box"
i_3rd_box_toggle = input.bool(defval=false, title="Third Box On/Offββββ", group=G3, inline="third_toggle")
i_3rd_box_bgcolor = input.color(defval=color.new(#7b1fa2, 80), title="Fill Color", group=G3, inline="third_toggle")
i_3rd_box_start_day = input.string(defval="America/New_York", title="Start Day Timezone", options=["America/New_York", "Europe/London", "Asia/Shanghai"], group=G3, tooltip=c_tooltip_2)
i_3rd_box_session = input.session(defval=c_new_york_killzone_est, title="Box Session Times", group=G3, tooltip=c_tooltip_3)
i_3rd_box_text = input.string(defval="3rd Box", title="Box Text Label", group=G3)
i_3rd_box_border_color = input.color(defval=color.new(#131722, 80), title="Borderβββββββ", group=G3, inline="third_border")
i_3rd_box_border_width = input.int(defval=2, title="", minval=1, maxval=20, group=G3, inline="third_border")
i_3rd_box_border_style = input.string(defval="solid (β)", title="", options=["solid (β)", "dotted (β)", "dashed (β)"], group=G3, inline="third_border")
// Text in all boxes
var string GT = "Box Text (All Boxes)"
i_box_text_color = input.color(defval=color.new(#131722, 20), title="βText Styleβ", group=GT, inline="ny_session3")
i_box_text_size = input.string(defval="Normal", title="", options=["Tiny", "Small", "Normal", "Large", "Huge", "Auto"], group=GT, inline="ny_session3")
i_box_textv_v = input.string(defval="Top", title="βText Position", options=["Top", "Center", "Bottom"], group=GT, inline="ny_session4")
i_box_text_h = input.string(defval="Left", title="", options=["Left", "Center", "Right"], group=GT, inline="ny_session4")
var string L1 = "Horizontal Line"
i_1st_line_toggle = input.bool(defval=false, title="Line On/Off", group=L1)
i_1st_line_label_toggle = input.bool(defval=false, title="label On/Off", group=L1)
i_1st_line_start_day = input.string(defval="America/New_York", title="Line Start Day Timezone", options=["America/New_York", "Europe/London", "Asia/Shanghai"], group=L1, tooltip=c_tooltip_2)
i_1st_line_session = input.session(defval='0100-1659', title="Line Start & End Times", group=L1, tooltip=c_tooltip_3)
i_1st_line_color = input.color(defval=color.new(#b22833, 20), title="Line & Line label Color", group=L1)
i_1st_line_style = input.string(defval="solid (β)", title="Style", options=["solid (β)", "dotted (β)", "dashed (β)"], group=L1, inline="line")
i_1st_text_color = input.string(defval="White", title="", options=["Black", "White"], group=L1, inline="line")
i_1st_line_width = input.int(defval=2, title="", minval=1, maxval=20, group=L1, inline="line")
//------------------------------------
// VARIABLES
//------------------------------------
// See if a new calendar day started on the intra-day time frame
// with variable timezone
newDayStartBox1 = dayofmonth(time, i_1st_box_start_day) != dayofmonth(time, i_1st_box_start_day)[1] and timeframe.isintraday
newDayStartBox2 = dayofmonth(time, i_2nd_box_start_day) != dayofmonth(time, i_2nd_box_start_day)[1] and timeframe.isintraday
newDayStartBox3 = dayofmonth(time, i_3rd_box_start_day) != dayofmonth(time, i_3rd_box_start_day)[1] and timeframe.isintraday
newDayStartLine = dayofmonth(time, i_1st_line_start_day) != dayofmonth(time, i_1st_line_start_day)[1] and timeframe.isintraday
///////////////////////////////////////////////////////////////////////////////// DAYS BACK
// Find the last bar on the chart
lastBarDate = timestamp(year(timenow), month(timenow), dayofmonth(timenow), hour(timenow), minute(timenow), second(timenow))
// Find the current bar that is being processed
thisBarDate = timestamp(year, month, dayofmonth, hour, minute, second)
// Find the number of days between these two bars
daysLeft = math.floor((lastBarDate - thisBarDate) / c_milliseconds_in_day)
// Only display the number of days specified in Input Settings
in_days_back_range = daysLeft < i_display_days_back
///////////////////////////////////////////////////////////////////////////////// HELPER FUNCTIONS
// Function the converts string inputs to line style code
line_style_function(input_var) =>
switch input_var
"dotted (β)" => line.style_dotted
"dashed (β)" => line.style_dashed
=> line.style_solid
// Function that converts the words black/white to their code equivalents
text_color_function(string_color) =>
switch string_color
"Black" => color.black
"White" => color.white
=> color.black
// Function that converts string inputs to text size code
text_size_function(string_size) =>
switch string_size
"Tiny" => size.tiny
"Small" => size.small
"Normal" => size.normal
"Large" => size.large
"Huge" => size.huge
"Auto" => size.auto
// Function that converts string inputs to text vertical alignment code
text_vertical_alignment_function(string_v) =>
switch string_v
"Top" => text.align_top
"Center" => text.align_center
"Bottom" => text.align_bottom
// Function that converts string inputs to text horizontal alignment code
text_horizontal_alignment_function(string_h) =>
switch string_h
"Left" => text.align_left
"Center" => text.align_center
"Right" => text.align_right
///////////////////////////////////////////////////////////////////////////////// Future Session Boxes
// Text functions for box variables
box_text_size = text_size_function(i_box_text_size)
box_textv_v = text_vertical_alignment_function(i_box_textv_v)
box_text_h = text_horizontal_alignment_function(i_box_text_h)
//------------------------------------
// 1st BOX SESSION
//------------------------------------
// Time period variables
first_box_session_est_window = time(timeframe.period, i_1st_box_session, "America/New_York")
// Parse start hour and start minutes from session string
first_box_start_hour = str.substring(i_1st_box_session, 0, 2)
first_box_start_minute = str.substring(i_1st_box_session, 2, 4)
// Use time hour and minute to create boolean for time
first_box_time_start = (hour(time, "America/New_York") == str.tonumber(first_box_start_hour)) and (minute(time) == str.tonumber(first_box_start_minute))
// Text functions for box variables
first_box_border_style = line_style_function(i_1st_box_border_style)
// Box defaults
var box first_box = na
var float first_high = 0.0
var float first_low = 0.0
// Only display the specified number of days back
if in_days_back_range
// Toggle NY Session box on/off
// Only display when timeframe is 30 minutes or less
if i_1st_box_toggle and timeframe.isminutes and timeframe.multiplier < 60
// If a new day starts, set the high and low to that bar's data. Else
// during the day track the highest high and lowest low.
if newDayStartBox1
// On start of new day create a blank box
first_box := box.new(left=na, top=na, right=na, bottom=na, xloc=xloc.bar_index, text=i_1st_box_text, text_color=i_box_text_color, text_halign=box_text_h, text_valign=box_textv_v, text_size=box_text_size, border_color=i_1st_box_border_color, border_width=i_1st_box_border_width, border_style=first_box_border_style, bgcolor=i_1st_box_bgcolor)
else
// At specified time calculate highs and lows. Draw first box
if first_box_time_start
first_high := high
first_low := low
box.set_left(first_box, bar_index)
box.set_top(first_box, first_high)
box.set_right(first_box, bar_index + 1)
box.set_bottom(first_box, first_low)
// At every new candle during 1st Box timespan update highs and lows and redraw the box
if first_box_session_est_window
first_high := math.max(first_high, high)
first_low := math.min(first_low, low)
box.set_right(first_box, bar_index)
box.set_bottom(first_box, first_low)
box.set_top(first_box, first_high)
//------------------------------------
// 2nd BOX SESSION
//------------------------------------
// Time period variables
second_box_session_est_window = time(timeframe.period, i_2nd_box_session, "America/New_York")
// Parse start hour and start minutes from session string
second_box_start_hour = str.substring(i_2nd_box_session, 0, 2)
second_box_start_minute = str.substring(i_2nd_box_session, 2, 4)
// Use time hour and minute to create boolean for time
second_box_time_start = (hour(time, "America/New_York") == str.tonumber(second_box_start_hour)) and (minute(time) == str.tonumber(second_box_start_minute))
// Text functions for box variables
second_box_border_style = line_style_function(i_2nd_box_border_style)
// Box defaults
var box second_box = na
var float second_high = 0.0
var float second_low = 0.0
// Only display the specified number of days back
if in_days_back_range
// Toggle 2nd Box Session box on/off
// Only display when timeframe is 30 minutes or less
if i_2nd_box_toggle and timeframe.isminutes and timeframe.multiplier < 60
// If a new day starts, set the high and low to that bar's data. Else
// during the day track the highest high and lowest low.
if newDayStartBox2
// On start of new day create a blank box
second_box := box.new(left=na, top=na, right=na, bottom=na, xloc=xloc.bar_index, text=i_2nd_box_text, text_color=i_box_text_color, text_halign=box_text_h, text_valign=box_textv_v, text_size=box_text_size, border_color=i_2nd_box_border_color, border_width=i_2nd_box_border_width, border_style=second_box_border_style, bgcolor=i_2nd_box_bgcolor)
else
// At specified time start calculate highs and lows. Draw first box
if second_box_time_start
second_high := high
second_low := low
box.set_left(second_box, bar_index)
box.set_top(second_box, second_high)
box.set_right(second_box, bar_index + 1)
box.set_bottom(second_box, second_low)
// At every new candle during 2nd Box Session update highs and lows and redraw the box
if second_box_session_est_window
second_high := math.max(second_high, high)
second_low := math.min(second_low, low)
box.set_right(second_box, bar_index)
box.set_bottom(second_box, second_low)
box.set_top(second_box, second_high)
//------------------------------------
// 3rd BOX SESSION
//------------------------------------
// Time period variables
third_box_session_est_window = time(timeframe.period, i_3rd_box_session, "America/New_York")
// Parse start hour and start minutes from session string
third_box_start_hour = str.substring(i_3rd_box_session, 0, 2)
third_box_start_minute = str.substring(i_3rd_box_session, 2, 4)
// Use time hour and minute to create boolean for time
third_box_time_start = (hour(time, "America/New_York") == str.tonumber(third_box_start_hour)) and (minute(time) == str.tonumber(third_box_start_minute))
// Text functions for box variables
third_box_border_style = line_style_function(i_3rd_box_border_style)
// Box defaults
var box third_box = na
var float third_high = 0.0
var float third_low = 0.0
// Only display the specified number of days back
if in_days_back_range
// Toggle NY Session box on/off
// Only display when timeframe is 30 minutes or less
if i_3rd_box_toggle and timeframe.isminutes and timeframe.multiplier < 60
// If a new day starts, set the high and low to that bar's data. Else
// during the day track the highest high and lowest low.
if newDayStartBox3
// On start of new day create a blank box
third_box := box.new(left=na, top=na, right=na, bottom=na, xloc=xloc.bar_index, text=i_3rd_box_text, text_color=i_box_text_color, text_halign=box_text_h, text_valign=box_textv_v, text_size=box_text_size, border_color=i_3rd_box_border_color, border_width=i_3rd_box_border_width, border_style=third_box_border_style, bgcolor=i_3rd_box_bgcolor)
else
// At specified time calculate highs and lows. Draw first box
if third_box_time_start
third_high := high
third_low := low
box.set_left(third_box, bar_index)
box.set_top(third_box, third_high)
box.set_right(third_box, bar_index + 1)
box.set_bottom(third_box, third_low)
// At every new candle during 1st Box timespan update highs and lows and redraw the box
if third_box_session_est_window
third_high := math.max(third_high, high)
third_low := math.min(third_low, low)
box.set_right(third_box, bar_index)
box.set_bottom(third_box, third_low)
box.set_top(third_box, third_high)
///////////////////////////////////////////////////////////////////////////////// HORIZONTAL TIME LINE
// Time period variables
first_line_session_est_window = time(timeframe.period, i_1st_line_session, "America/New_York")
// Parse start hour and start minutes from session string
first_line_start_hour = str.substring(i_1st_line_session, 0, 2)
first_line_start_minute = str.substring(i_1st_line_session, 2, 4)
// Use time hour and minute to create boolean for time
first_line_time_start = (hour(time, "America/New_York") == str.tonumber(first_line_start_hour)) and (minute(time) == str.tonumber(first_line_start_minute))
first_line_style = line_style_function(i_1st_line_style)
first_line_text_color = text_color_function(i_1st_text_color)
// Line defaults
var line line_first = na
var label label_first = na
var float price_first_line = na
// Only display the specified number of days back
if in_days_back_range
// Draw line starting at the midnight open EST candle
if i_1st_line_toggle and timeframe.isminutes and timeframe.multiplier < 60
// If a new day starts, set the high and low to that bar's data. Else
// during the day track the highest high and lowest low.
// Used London new day time because NY new day is the time we are looking for. Using NY new day wouldn't work.
if newDayStartLine
// On new day create blank line but with specified style
line_first := line.new(x1=na, y1=na, x2=na, y2=na, color=i_1st_line_color, style=first_line_style, width=i_1st_line_width)
else
// At exactly midnight EST
if first_line_time_start
// Update line with current x's and y's
line.set_x1(line_first, bar_index)
line.set_y1(line_first, open)
line.set_x2(line_first, bar_index + 1)
line.set_y2(line_first, open)
// At every new candle during specified time span, update x2 value to move the line to the right with the current bar
if first_line_session_est_window
line.set_x2(line_first, bar_index)
// Draw label only for most recent line
if i_1st_line_label_toggle
// Get y value (price) of midnight open line
price_first_line := line.get_price(line_first, bar_index)
label_first := label.new(x=bar_index + 5, y=price_first_line, text=str.tostring(price_first_line), xloc=xloc.bar_index, color=i_1st_line_color, textcolor=first_line_text_color, tooltip=str.tostring(i_1st_line_session))
label.delete(label_first[1])
/////////////////////////////////////////////////////////////////////////////////